LARAVEL FOR DUMMIES – THE ULTIMATE GUIDE
- Category : Server Administration
- Posted on : Mar 16, 2018
- Views : 1,946
- By : Tadashi P.
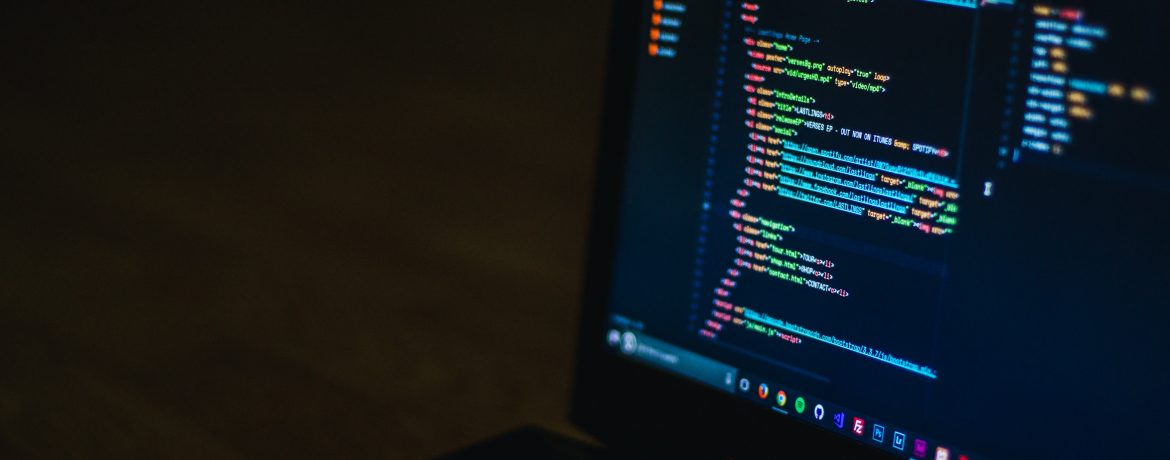
PHP is a programming language that remains by far the top choice for web development. While it is criticized for not being a clean language or a very fast one, the latest statistics show that almost 80% of all the websites in the world are PHP-based.
It is used everywhere and provides the best compatibility, since every hosting platform supports PHP. This is not always the case with more advanced web development languages, such as Ruby or Python.
ADVANTAGES OF LARAVEL
Coders rarely write web applications from scratch, since frameworks provide a very solid structure to any new project and considerably speed up development time.
Several high quality frameworks are available for PHP and Laravel is by far the most widely used of them, with over 51000 stars on its Github repository.
There are many reasons for this popularity: Laravel is open-source, with a very fast engine and the most extensive documentation of all frameworks. It can effectively handle all of the common functions of a modern website, such as authentication, routing, session management or caching.
Laravel integrates easily with mail systems, offers built-in testing and is highly scalable. In addition, it is constantly updated in order to use the newest features of PHP and its code is very secure, protecting web applications against the most dangerous security risks.
MAIN LARAVEL COMPONENTS
Laravel provides many components and features that simplify the tasks of a web developer. One of the most important is integration with the Composer package management tool, which makes it very easy to install additional libraries and dependencies as bundles.
Database data is accessed through the included query builder but the framework also provides Eloquent ORM, an active record implementation that defines the relation between database-type objects.
In order to build dynamic blocks from predefined layouts, Laravel uses the advanced Blade engine, which is highly customizable and has its own loops and conditional statements.
All of the common authentication features are already built in the framework, handling services such as registration or lost passwords. For caching, Laravel uses Redis for both session management and to optimize loading times.
Other advanced functions are available by default in Laravel, so external services are not needed. These include the Migrations version control system, as well as integrated unit testing or class auto loading, which makes sure that only the used components are loaded.
Laravel also includes an integrated command line tool named Artisan that provides helpful commands for web application automation.
LARAVEL PREREQUISITES
Laravel can be installed on a wide range of operating systems, in this tutorial we will use a clean CentOS 7 server, with all packages updated to the latest version.
The framework needs a functional LAMP stack, which uses Apache as the web server, as well as PHP and MySQL. We will not detail the installation of these services, but our server runs Apache 2.4, MySQL 5.7 and PHP 7.2. Besides PHP, we have also installed a number of common modules such as mbstring, zip, xml or mcrypt, as well as the modules actually required by Laravel (OpenSSL, pdo, tokenizer, ctype, json and bcmath).
INSTALL COMPOSER
The Composer package manager is used by Laravel to manage dependencies but can also be used to install the framework itself.
The latest version of Composer can be downloaded with curl:
1 | curl -sS https://getcomposer.org/installer | php |
An advantage of this method is that the Composer installer automatically checks if all settings are correct and the prerequisites are met, before configuring the package.
1 2 | mv composer.phar /usr/bin/composer chmod +x /usr/bin/composer |
You can now move the binary file to the proper location and make it executable, so it can be used as a system command.
If you run into any installation issues, execute the composer diagnose command. It will automatically check for the most common problems, as well as suggest fixes for them.
INSTALL LARAVEL
Laravel can be installed in multiple ways; the simplest one recommended by the framework’s documentation is to use the official installer.
Very conveniently, the installer can be easily executed using Composer:
1 | composer global require laravel/installer |
You’ll notice that the installer changes the working directory to /root/.config/composer (when executed as root). In order for Laravel to work properly, the vendor bin folder location must be in your global $PATH environment variable:
1 | PATH=$PATH:/root/.config/composer/vendor/bin |
Paste this line in the ~/.profile file, so that the path will be setup correctly after every system reboot.
That was it, Laravel is now installed and functional. You can use the binary command to create a new installation of the framework in any desired folder.
For example, navigate to the default website root folder of the Apache webserver and start a new Laravel installation in a folder named blog:
1 2 | cd /var/www/html laravel new blog |
If everything went well, the last two lines of the output should look like this:
Package manifest generated successfully.
Application ready! Build something amazing.
ALTERNATIVE INSTALLATION METHODS
If you just want to test Laravel, the easiest way to do it is to use Homestead, which is an official Vagrant box that can quickly be installed as a virtual machine on all major operating systems.
It provides a fully functional installation, with all services already configured. Another advantage is that virtual machines are disposable, so you can start a new one in just a few minutes, as you learn how to use the framework.
Another install method that doesn’t use the official installation script is directly from Composer, using the create-project command.
Finally, you can try Laravel using the development server already available in php, by executing the following command:
1 | php artisan serve |
The development server runs on the localhost port 8000 and can be accessed in your local browser. Of course, this option should not be used in a production environment.
CONFIGURE THE FRAMEWORK
The document root of your web server should now point to Laravel’s public directory.
If you’re using an actual domain, you can configure a Virtual Host block in Apache for this purpose. Otherwise, you can simply change the default document root line in the file /etc/httpd/conf/httpd.conf:
1 | DocumentRoot "/var/www/html/blog/public" |
Apache must be restarted in order for the change to take effect.
The next step is fixing folder permissions. If you installed Laravel as root, as in the example above, the webserver will not have the correct write rights.
The easiest option in this case is to recursively change the ownership of the entire blog folder to apache:apache. At the very least, Apache needs write permissions to all of the folders in the structure of the storage and bootstrap/cache paths.
After configuring the document root and fixing permissions, the mail page of your application should look like this:
All the configuration files are conveniently located in the config folder. Feel free to take a look in these files, every option is very well documented and you can easily find additional online resources that explain them in depth.
The most common options that have to be changed are typically the timezone and locale values, found in the app.php configuration file.
The final mandatory step recommended by the developers is to make sure that your application key is set to a random string. If you installed Laravel through Composer or the official script, a random key has already been generated for you and you can find it in the .env hidden file in the project’s root.
Having a random key is very important in order to encrypt very sensitive data such as user sessions and protect user privacy.
Congratulations, you now have a fully functional Laravel project. You should now tweak other settings, such as database and caching options. We will not describe these settings because they are very well covered by both official and unofficial documentation sources.
The default installation of Laravel provides a .htaccess file in the public folder that rewrites URLs in order to make them prettier for the end user.
The rules in this file should work by default, as long as the mod_rewrite module is enabled in Apache. You can test this easily with the following command, the output should be “rewrite_module (shared)”:
1 | httpd -M | grep rewrite |
INSTALL LARAVEL BACKPACK
In order to make your Laravel projects even more interactive and powerful, we will also install and configure Laravel Backpack.
The basic package provides a visual interface for Laravel that simplifies many tasks such as authentication and notifications. Installing further packages allows you to actually build complex administration panels for your applications.
Before proceeding, you must make sure that you create a user and database for your project in MySQL, and then insert the correct credentials in your .env file.
To create the user and database, run the following commands from the mysql prompt:
1 2 3 4 | create database database_name; create user 'user_name'@'localhost' identified by 'strong_password'; grant all privileges on database_name.* to 'user_name'@'localhost'; flush privileges; |
The base Backpack module can now be installed using Composer:
1 2 | composer require backpack/base php artisan backpack:base:install |
You can now access the demo admin panel in your browser at <IP_or_domain>/ vendor/adminlte/
The next module to install is backpack/CRUD:
1 | composer require backpack/crud |
Run the following commands in order to create the uploads folder and publish various required files:
1 2 3 4 5 6 | mkdir public/uploads php artisan elfinder:publish php artisan vendor:publish --provider="BackpackCRUDCrudServiceProvider" --tag="public" php artisan vendor:publish --provider="BackpackCRUDCrudServiceProvider" --tag="lang" php artisan vendor:publish --provider="BackpackCRUDCrudServiceProvider" --tag="config" php artisan vendor:publish --provider="BackpackCRUDCrudServiceProvider" --tag="elfinder" |
Edite the config/filesystems.php and add this block to define the uploads disk:
1 2 3 4 | 'uploads' => [ 'driver' => 'local', 'root' => public_path('uploads'), ], |
INSTALL ADDITIONAL BACKPACK MODULES
All of the other Backpack modules are optional, there are many of these and there is no need to install them unless they are needed for your project.
For the purpose of this tutorial, we will install and setup the three most common ones.
The first of these is the langfilemanager:
1 | composer require backpack/langfilemanager |
The next commands are needed to configure the package and publish its files:
1 2 3 4 | php artisan migrate --path=vendor/backpack/langfilemanager/src/database/migrations php artisan db:seed --class="BackpackLangFileManagerdatabaseseedsLanguageTableSeeder" php artisan vendor:publish --provider="BackpackLangFileManagerLangFileManagerServiceProvider" --tag="config" php artisan vendor:publish --provider="BackpackLangFileManagerLangFileManagerServiceProvider" --tag="lang" |
The backup manager is another very useful module, the installation is again very easy:
1 2 | composer require backpack/backupmanager php artisan vendor:publish --provider="BackpackBackupManagerBackupManagerServiceProvider" |
Edit the config/filesystems.php file and add a new block to define a disk:
1 2 3 4 | 'backups' => [ 'driver' => 'local', 'root' => storage_path('backups'), // that's where your backups are stored by default: storage/backups ], |
This module is compatible with the most common cloud storage providers, so you can define the driver to store your data in another location, such as Amazon S3, Google Cloud or Dropbox.
More detailed backup options can be configured in the config/laravel-backup.php file, while in the app/Console/Kernel.php file you can define a function to schedule automatic backups.
The last optional Backpack module that we’re going to install is the log manager:
1 | composer require backpack/logmanager |
Similar to the other modules, the config/filesystems.php must be edited to define a filesystem by adding this block:
1 2 3 4 | 'storage' => [ 'driver' => 'local', 'root' => storage_path(), ], |
The default Backpack setting is to rotate the log file daily, so only one will be available. If you want to have a daily log file, add this line in your .env settings:
1 | APP_LOG=daily |
The same setting can be configured in the config/app.php file as well.
LEARNING THE FRAMEWORK
There are many excellent tutorials available to learn Laravel, but probably the best option is the Laravel From Scratch series that can be found on the official website.
It is a free series of videos, split into 38 episodes, which are designed for new users. They are constantly updated in order to reflect the latest Laravel features. Completing the series can be an excellent starting point in your career as a developer.
FINAL CONSIDERATIONS
Laravel is a very powerful php framework and mastering it can be extremely rewarding.
In this tutorial, we have presented the main features of the framework, as well as the installation of all components required to run it. In addition, the graphical interface Backpack greatly simplifies the flow of creating a new project.
Happy coding!
Categories
Subscribe Now
10,000 successful online businessmen like to have our content directly delivered to their inbox. Subscribe to our newsletter!Archive Calendar
Sat | Sun | Mon | Tue | Wed | Thu | Fri |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | |||
5 | 6 | 7 | 8 | 9 | 10 | 11 |
12 | 13 | 14 | 15 | 16 | 17 | 18 |
19 | 20 | 21 | 22 | 23 | 24 | 25 |
26 | 27 | 28 | 29 | 30 | 31 |
Recent Articles
-
Posted on : Jul 25
-
Posted on : Jul 07
-
Posted on : Apr 07
-
Posted on : Mar 19
Optimized my.cnf configuration for MySQL 8 (on cPanel/WHM servers)
Tags
- layer 7
- tweak
- kill
- process
- sql
- Knowledge
- vpn
- seo vpn
- wireguard
- webmail
- ddos mitigation
- attack
- ddos
- DMARC
- server load
- Development
- nginx
- php-fpm
- cheap vpn
- Hosting Security
- xampp
- Plesk
- cpulimit
- VPS Hosting
- smtp
- smtp relay
- exim
- Comparison
- cpu
- WHM
- mariadb
- encryption
- sysstat
- optimize
- Link Building
- apache
- centos
- Small Business
- VPS
- Error
- SSD Hosting
- Networking
- optimization
- DNS
- mysql
- ubuntu
- Linux